Face detection using TinyFace¶
This package comes with a TinyFace face detector. The Original Model is ResNet101
from tinyface. Please check for more details on TinyFace. The
model is converted into MxNet Interface and the code used to implement the model are
from hr101_mxnet.
Implementation¶
See below for an example on how to use bob.ip.facedetect.tinyface.TinyFacesDetector
:
1import matplotlib.pyplot as plt
2from bob.io.base import load
3from bob.io.base.test_utils import datafile
4from bob.io.image import imshow
5from bob.ip.facedetect.tinyface import TinyFacesDetector
6from matplotlib.patches import Rectangle
7
8# load colored test image
9color_image = load(datafile("test_image_multi_face.png", "bob.ip.facedetect"))
10is_mxnet_available = True
11try:
12 import mxnet
13except Exception:
14 is_mxnet_available = False
15
16if not is_mxnet_available:
17 imshow(color_image)
18else:
19
20 # detect all faces
21 detector = TinyFacesDetector()
22 detections = detector.detect(color_image)
23
24 imshow(color_image)
25 plt.axis("off")
26
27 for annotations in detections:
28 topleft = annotations["topleft"]
29 bottomright = annotations["bottomright"]
30 size = bottomright[0] - topleft[0], bottomright[1] - topleft[1]
31 # draw bounding boxes
32 plt.gca().add_patch(
33 Rectangle(
34 topleft[::-1],
35 size[1],
36 size[0],
37 edgecolor="b",
38 facecolor="none",
39 linewidth=2,
40 )
41 )
This face detector can be used for detecting single or multiple faces. If there are more than one face, the first entry of the returned annotation supposed to be the largest face in the image.
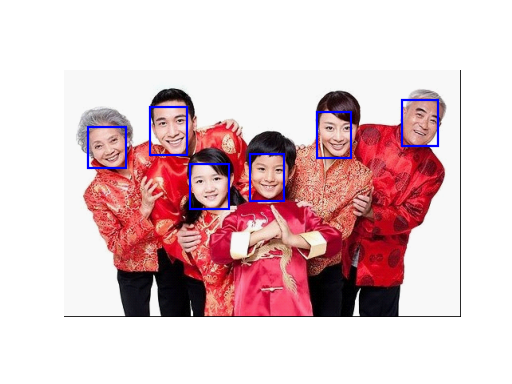
Fig. 1 Multiple faces are detected by TinyFace.¶